Swift 5.9 introduced if
and switch
expressions that allow you to write shorter code by omitting the return keyword. The feature resembles Swift 5.1’s ability to omit the return keyword in single expressions inside closures.
While shorter code doesn’t necessarily always lead to more readable code, omitting return keywords inside if
and switch
expressions certainly does. Your code becomes easier and more natural to read, creating a win-win outcome.
Using if statements as expressions
You can use if
statements as expressions to reduce the line of code required for an assignment. For example, imagine setting a text color based on whether dark mode is active:
let textColor: Color
if isDarkMode {
textColor = .white
} else {
textColor = .black
}
This is readable and works great, but takes six lines of code. That might be fine, but combining many statements could easily create long function bodies.
You might be considering to use the ternary operator instead:
let textColor: Color = isDarkMode ? .white : .black
However, many developers find it hard to read ternary operator statements.
Swift 5.9 allows you to write the same code using if statements as expressions:
let textColor: Color = if isDarkMode { .white } else { .black }
Its behavior is similar to omitting the return keyword and requires the if statement branch to be a single expression. In other words, you can’t put multiple lines of code inside a single branch. Note that we could write the above code over multiple lines as well:
let textColor: Color = if isDarkMode {
.white
} else {
.black
}
Lastly, you can’t use if statements as expressions without an else statement:
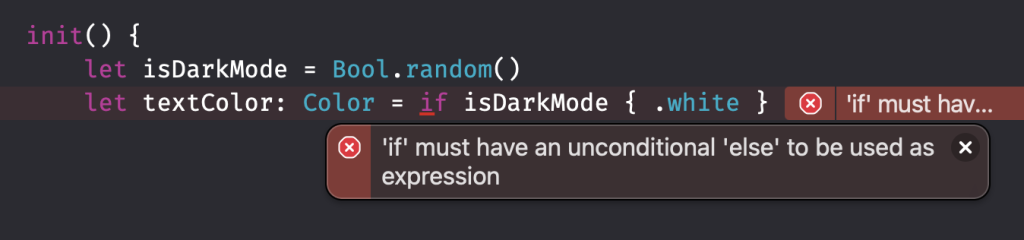
Using switch statements as expressions
When writing switch statements in Swift, you might have often written code like this:
enum Subscription {
case free, pro, premium
var title: String {
switch self {
case .free:
return "Free"
case .pro:
return "Pro"
case .premium:
return "Premium"
}
}
}
While it’s readable code, writing the return keyword on a new line for every case feels a bit redundant. Before Swift 5.9, we could already shorten this code as follows:
var title: String {
switch self {
case .free: return "Free"
case .pro: return "Pro"
case .premium: return "Premium"
}
}
Now, in Swift 5.9, we can further reduce the code used and omit the return keyword as well:
var title: String {
switch self {
case .free: "Free"
case .pro: "Pro"
case .premium: "Premium"
}
}
Conclusion
Using if
and switch
statements as expressions can reduce the lines of code you write while maintaining readability. Like omitting the return keyword inside closures, you can now omit the keyword inside single-expression if and switch statements.
If you like to improve your Swift knowledge, even more, check out the Swift category page. Feel free to contact me or tweet to me on Twitter if you have any additional tips or feedback.
Thanks!