“The operation couldn’t be completed” is a common error to receive from Apple’s standard SDKs or 3rd party libraries. The errors often come with an error code that doesn’t have a description, leaving you behind with unclear directions on solving the issue.
I’ve been running into these errors quite often, at which I opened several gist’s on GitHub to find out the description behind an error code. Objective-C header files with partly documented static keys give a vague indication of the thrown error. Let’s dive into these errors and see how you can get solutions.
If you’re new to error handling in Swift, I encourage you first to read Try Catch Throw: Error Handling in Swift with Code Examples.
Understanding the format of thrown errors
Operations that couldn’t complete often result in errors looking as follows:
Error Domain=NSURLErrorDomain Code=-1012 "The operation couldn’t be completed. (NSURLErrorDomain error -1012.)"
UserInfo=0x20166b722 {
NSErrorFailingURLStringKey=https://someurl,
NSUnderlyingError=0x20166b722 "The operation couldn’t be completed. (kCFErrorDomainCFNetwork error -1012.)",
NSErrorFailingURLKey=https://someurl
}
We know it’s some URL-related error with a -1012
code, but we don’t get any description of this error code. The user info contains hints like CFNetwork (the underlying framework that performed the request) and the related URL we executed. However, we must open our browsers and search for answers.
Finding descriptions for error codes
For most error domains, gist’s on GitHub will help you find a description of the error code. Searching for NSURLErrorDomain codes
leads us to this gist as an example. On that page, we’ll find the following list of error keys and their related codes:
kCFURLErrorUnknown = -998,
kCFURLErrorCancelled = -999,
kCFURLErrorBadURL = -1000,
kCFURLErrorTimedOut = -1001,
kCFURLErrorUnsupportedURL = -1002,
kCFURLErrorCannotFindHost = -1003,
kCFURLErrorCannotConnectToHost = -1004,
kCFURLErrorNetworkConnectionLost = -1005,
kCFURLErrorDNSLookupFailed = -1006,
kCFURLErrorHTTPTooManyRedirects = -1007,
kCFURLErrorResourceUnavailable = -1008,
kCFURLErrorNotConnectedToInternet = -1009,
kCFURLErrorRedirectToNonExistentLocation = -1010,
kCFURLErrorBadServerResponse = -1011,
kCFURLErrorUserCancelledAuthentication = -1012,
kCFURLErrorUserAuthenticationRequired = -1013,
kCFURLErrorZeroByteResource = -1014,
kCFURLErrorCannotDecodeRawData = -1015,
kCFURLErrorCannotDecodeContentData = -1016,
kCFURLErrorCannotParseResponse = -1017,
kCFURLErrorInternationalRoamingOff = -1018,
kCFURLErrorCallIsActive = -1019,
kCFURLErrorDataNotAllowed = -1020,
kCFURLErrorRequestBodyStreamExhausted = -1021,
kCFURLErrorFileDoesNotExist = -1100,
kCFURLErrorFileIsDirectory = -1101,
kCFURLErrorNoPermissionsToReadFile = -1102,
kCFURLErrorDataLengthExceedsMaximum = -1103,
While this list isn’t complete, it does give us the following match for the -1012
code:
kCFURLErrorUserCancelledAuthentication = -1012
Our error code tells us the operation couldn’t be completed due to the user canceling the authentication.
Searching on Google for the relevant key
The next step will be to search for the matching key on Google. In this case, we’re searching for kCFURLErrorUserCancelledAuthentication
that will lead to the following Apple documentation:
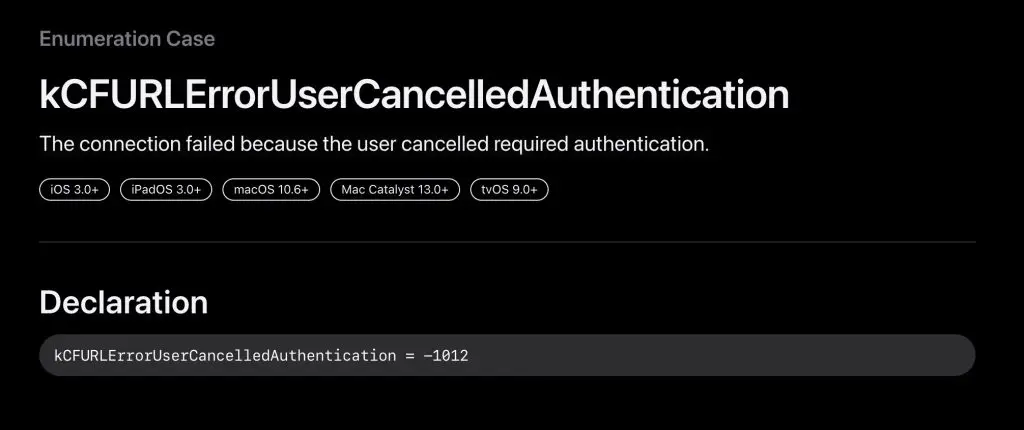
You can see the same -1012
error code, but this time it’s joined with a description:
The connection failed because the user cancelled required authentication.
These descriptions will often be enough to continue our journey into solving the occurred errors. However, there’s a way to speed up getting to an explanation.
Introducing What The Error Code
Since I traveled the journey between Google, Stack Overflow, and Apple documentation a little too often, I decided to create a simple tool that gives you the description immediately. You can fill in the complete error description and the app will give us the explanation:
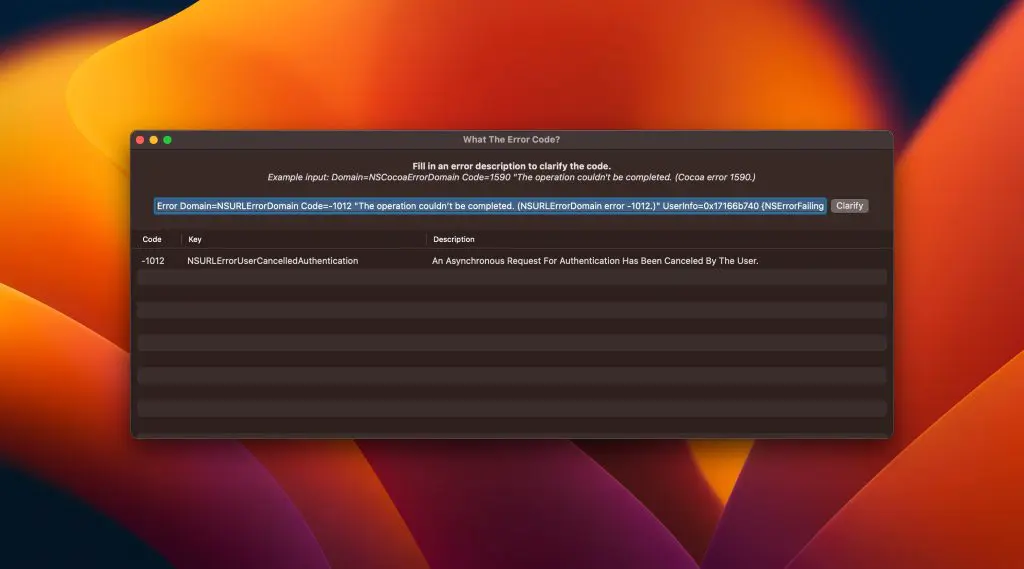
It’s a simple application backed by a list of error descriptions in this JSON file. A regex fetches the domain and code from the error description and matches it to the JSON keys to get an explanation.
Quick access using RocketSim Utilities
To simplify usage, I’ve added WhatTheErrorCode as a developer utility to RocketSim:
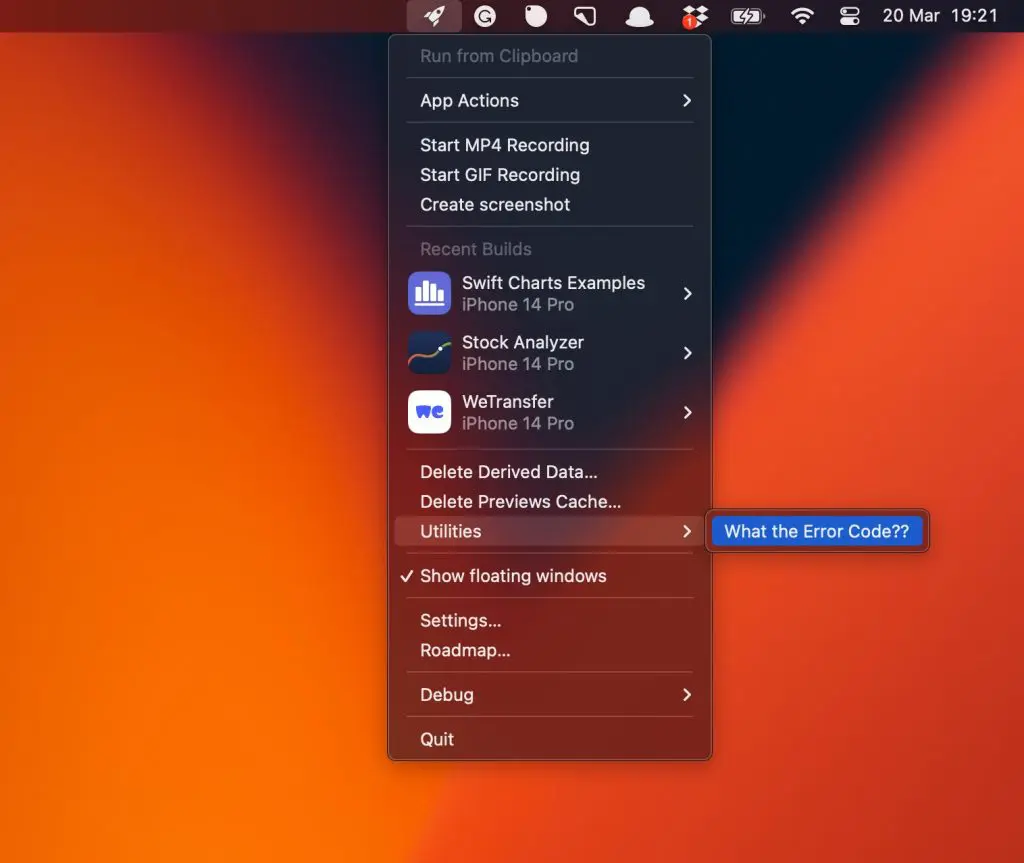
Conclusion
“The operation couldn’t be completed” is an annoying error description at first, but it can be converted into descriptions using the right technique. WhatTheErrorCode is an open-sourced alternative to speed up getting to explanations.
If you like to improve your Swift knowledge, even more, check out the Swift category page. Feel free to contact me or tweet to me on Twitter if you have any additional tips or feedback.
Thanks!